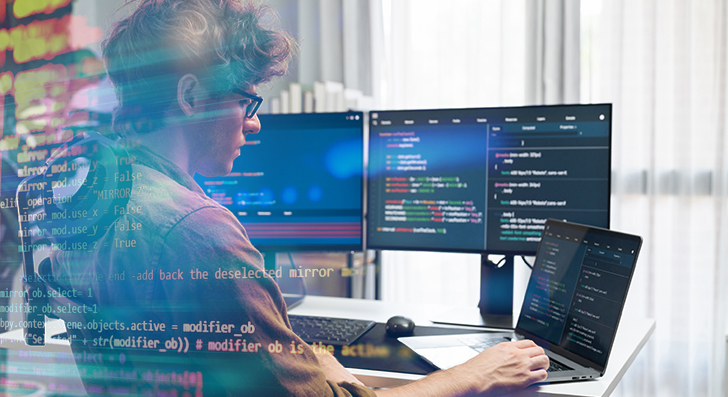
Scalability indicates your application can take care of progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the beginning. A lot of applications fall short when they increase fast mainly because the original style and design can’t take care of the additional load. As being a developer, you might want to Believe early regarding how your system will behave under pressure.
Get started by developing your architecture to generally be adaptable. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These designs split your application into smaller, unbiased parts. Every single module or company can scale on its own without having impacting The complete system.
Also, take into consideration your databases from day a single. Will it will need to take care of a million customers or maybe 100? Pick the ideal variety—relational or NoSQL—based upon how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them nonetheless.
Another essential position is to avoid hardcoding assumptions. Don’t create code that only functions below existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like information queues or celebration-pushed programs. These support your app cope with additional requests devoid of finding overloaded.
Any time you Make with scalability in mind, you're not just preparing for fulfillment—you might be reducing future problems. A perfectly-prepared program is easier to maintain, adapt, and mature. It’s superior to arrange early than to rebuild afterwards.
Use the ideal Databases
Selecting the correct database is usually a critical Section of creating scalable applications. Not all databases are crafted exactly the same, and utilizing the Mistaken one can slow you down or maybe lead to failures as your app grows.
Start out by comprehension your information. Can it be remarkably structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are potent with associations, transactions, and regularity. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional site visitors and details.
When your data is much more flexible—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and may scale horizontally extra very easily.
Also, think about your read through and write patterns. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy generate load? Consider databases that could tackle higher publish throughput, or simply event-primarily based knowledge storage units like Apache Kafka (for temporary facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them implies you received’t have to have to switch later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access patterns. And usually keep track of database efficiency when you improve.
To put it briefly, the ideal databases relies on your application’s composition, pace demands, And just how you assume it to increase. Choose time to select correctly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single modest hold off adds up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s essential to Create productive logic from the start.
Begin by crafting cleanse, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most advanced Remedy if a simple a person performs. Keep your capabilities short, centered, and simple to check. Use profiling equipment to locate bottlenecks—sites wherever your code will take too very long to run or takes advantage of excessive memory.
Following, take a look at your databases queries. These usually gradual items down more than the code by itself. Make certain Just about every query only asks for the information you actually will need. Keep away from SELECT *, which fetches almost everything, and rather decide on specific fields. Use indexes to speed up lookups. And stay clear of undertaking a lot of joins, Particularly throughout big tables.
When you notice a similar information staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to test with big datasets. Code and queries that perform high-quality with a hundred information may possibly crash if they have to take care of one million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software stay easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more end users and a lot more website traffic. If anything goes through one server, it will speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server undertaking each of the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to put in place.
Caching is about storing information quickly so it could be reused swiftly. When users ask for precisely the same info all over again—like an item web page or simply a profile—you don’t ought to fetch it in the databases when. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near to the person.
Caching decreases databases load, improves velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does adjust.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app tackle much more end users, continue to be quickly, and Get well from problems. If you plan to increase, you need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need equipment that allow your application grow very easily. That’s the place cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess long run potential. When targeted visitors increases, you'll be able to incorporate a lot more assets with just a couple clicks or routinely using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security applications. You could focus on building your application in place of taking care of infrastructure.
Containers are One more essential Device. A container packages your app and every thing it needs to operate—code, libraries, options—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app takes advantage of a number of containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it more info restarts it instantly.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
To put it briefly, making use of cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get better rapidly when complications take place. If you prefer your app to improve without having restrictions, commence applying these resources early. They help save time, decrease chance, and help you continue to be focused on creating, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. By way of example, When your response time goes over a limit or perhaps a services goes down, you should get notified immediately. This helps you fix challenges speedy, generally in advance of buyers even detect.
Checking is additionally helpful whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you could roll it back again just before it causes serious destruction.
As your app grows, visitors and data raise. With no monitoring, you’ll pass up signs of trouble right until it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for major businesses. Even compact apps will need a powerful Basis. By creating carefully, optimizing properly, and utilizing the correct applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Begin modest, think huge, and Establish intelligent.